External Filters for Kendo Grid
So What is Keno UI Grid?
Kendo UI Grid is a feature rich HTML5 Grid control provided as part of Telerik’s Kendo UI Web framework. Kendo Grid provides a lot of features out of the box, such as filtering, grouping, paging, sorting, and support for various data formats.
Filtering
The default filtering interface for Kendo Grid is very powerful. However, it is generic to accommodate for all kind of data that you can throw at it.
To give you a quick example, here are different filter interfaces for different data type:
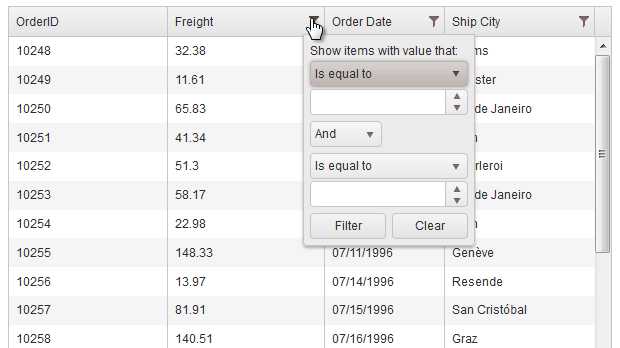
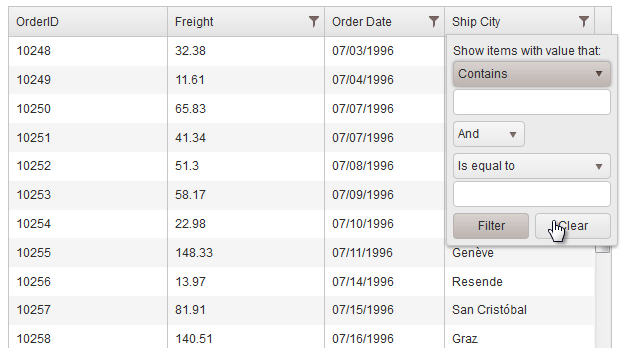
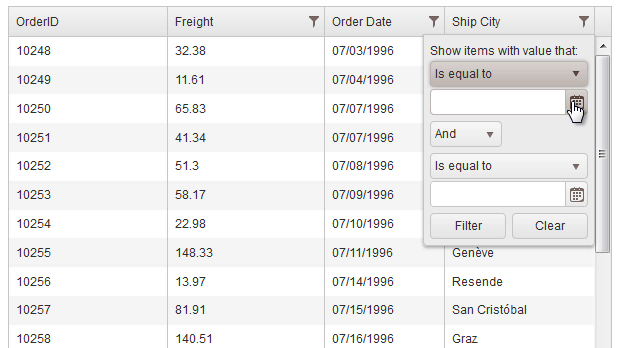
In my case, the built-in filtering interface was not desirable. There were couple of reasons for that.
- I didn’t want to retrain my users. A previous Web Forms based version of the application was already using dropdown boxes for filtering Telerik Rad Grid.
- The filtering interface, although very powerfull, can be confusing. My aim was to provide dropdown boxes, with distinct values for the users to filter on.
To The Code…
My scenario is very simple. I have a Machines table, which lists some machine names along with their operating system and the network they are on.
Network | OS | Hostname |
---|---|---|
A | Win 7 | W7-001 |
A | Win 8 | W8-002 |
B | Win 7 | W7-003 |
B | Win 8.1 | W81-004 |
B | Win 7 | W7-005 |
C | Redhat | RH-006 |
C | Win 7 | W7-007 |
C | Win 8.1 | W81-008 |
Grid
Setting up Kendo Grid is very simple. Here’s the code for my grid embedded in a Razor view:
1
2
3
4
5
6
7
8
9
10
11
12
13
@(Html.Kendo().Grid<MyPortal.Models.Machines>()
.Name("MachinesGrid")
.Columns(columns =>
{
columns.Bound(e => e.Network);
columns.Bound(e => e.OperatingSystem);
columns.Bound(e => e.Hostname);
})
.Sortable(sortable => sortable.AllowUnsort(false))
.DataSource(ds => ds
.Ajax()
.Read(read => read.Action("GetGridData", "Machine"))
));
For a simpler dataset, represented as an HTML table, you can use the following code to quickly initialize a grid around it.
1
2
3
4
// Initialize Kendo Grid from a table with the ID of "TableGrid".
$("#TableGrid").kendoGrid({
sortable: true
});
Manipulating the Grid
Kendo Grid provides a client side API, which you can leverage to access and manipulate the Grid object.
1
2
3
4
5
// accessing the Grid object
var grid = $("#MachinesGrid").data("kendoGrid");
// accessing the current filters
var currentFilterOjb = grid.dataSource.filter()
The currentFilterObj
contains logic
and filters
fields.
-
logic
specifies and or or logic for the filters. -
filters
specifies an array containing the individual filter objects.
External Filters
For my example, I have defined two dropdowns and one button. The dropdown will dynamically update the filter via change
event handler. The button will be used to clear all filters. I’ll skip the HTML code for the dropdowns and the button.
The javascript event handlers are defined below:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
// attache change event for "OS" dropdown
$("#os").change(function () {
applyFilter("OS", $(this).val());
});
// attach change event to the "network" dropdown.
$("#network").change(function () {
applyFilter("Network", $(this).val());
});
// attach click event for Clear Filters button
$("#clear").click(function () {
clearFilters();
});
Applying Filters
The following function takes a field name to filter on and a value for the filter. It applies a filter based on the following logic:
- Get currently applied filter(s) to the Grid
- If no filters are applied, created an empty array to hold new filter(s)
- Iterate through current filters, if a filter is found for the currently specified field, remove it.
- If the value is not
0
, create a new filter object and add it to the filters array. - apply the new filters to the grid.
This function will let you progressively apply multiple filters. It will also remove a single filter if the dummy value is chosen for the dropdown.
Here is the Javascript code to accomplish all this.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
// applyFilter function accepts the Field Name and the new value to use for filter.
function applyFilter(filterField, filterValue) {
// get the kendoGrid element.
var gridData = $("#MachineGrid").data("kendoGrid");
// get currently applied filters from the Grid.
var currFilterObj = gridData.dataSource.filter();
// get current set of filters, which is supposed to be array.
// if the oject we obtained above is null/undefined, set this to an empty array
var currentFilters = currFilterObj ? currFilterObj.filters : [];
// iterate over current filters array. if a filter for "filterField" is already
// defined, remove it from the array
// once an entry is removed, we stop looking at the rest of the array.
if (currentFilters && currentFilters.length > 0) {
for (var i = 0; i < currentFilters.length; i++) {
if (currentFilters[i].field == filterField) {
currentFilters.splice(i, 1);
break;
}
}
}
// if "filterValue" is "0", meaning "-- select --" option is selected, we don't
// do any further processing. That will be equivalent of removing the filter.
// if a filterValue is selected, we add a new object to the currentFilters array.
if (filterValue != "0") {
currentFilters.push({field: filterField,operator: "eq",value: filterValue});
}
// finally, the currentFilters array is applied back to the Grid, using "and" logic.
gridData.dataSource.filter({ logic: "and", filters: currentFilters });
}
The syntax for a new filter is:
1
{field: "field Name", operator: "eq", value: "value" }
This example uses eq
or equal
filter operation. You can read up on various different filter operators at the following Telerik Grid Filter Operators.
Clearing All Filters
The following simple function will clear all filters on the grid.
1
2
3
4
function clearFilters() {
var gridData = $("#MachinesGrid").data("kendoGrid");
gridData.dataSource.filter({});
}
Putting It All Together
I’ve put together a fully functional example with the functions referenced above, which uses a simple table as the grid data source.
You can take a look at the following JSFiddle to see the logic and code in action.
Kendo UI Grid - External Filter - http://jsfiddle.net/randombw/27hTK/